Simplified PHP Code to Send an FCM notification
Simplified PHP Code to Send an FCM notification using the https://fcm.googleapis.com/fcm/send endpoint with a title, body, and extra parameters
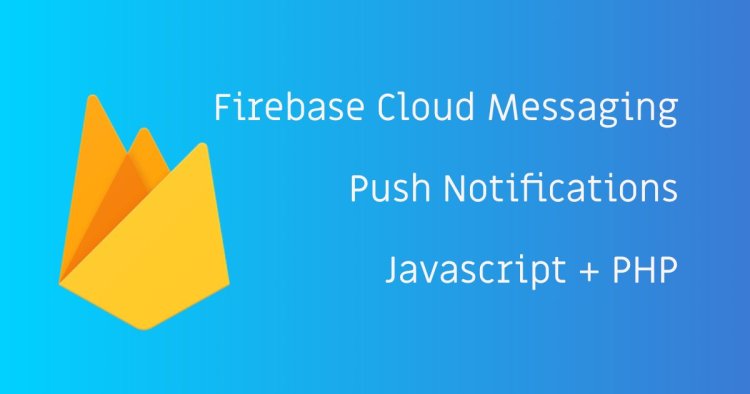
<?php
$serverKey = 'your_server_key';
$deviceToken = 'device_fcm_token';
$title = 'Notification Title';
$body = 'Notification Body';
$extraParameters = [
'parameter1' => 'Value1',
'parameter2' => 'Value2',
];
$data = [
'to' => $deviceToken,
'notification' => [
'title' => $title,
'body' => $body,
],
'data' => $extraParameters,
];
$jsonPayload = json_encode($data);
$headers = [
'Authorization: key=' . $serverKey,
'Content-Type: application/json',
];
$ch = curl_init('https://fcm.googleapis.com/fcm/send');
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonPayload);
$response = curl_exec($ch);
if (curl_errno($ch)) {
echo 'cURL error: ' . curl_error($ch);
} else {
$decodedResponse = json_decode($response);
if (isset($decodedResponse->success) && $decodedResponse->success == 1) {
echo 'Notification sent successfully';
} else {
echo 'Failed to send notification';
}
}
curl_close($ch);
?>
Simply replace 'your_server_key'
with your FCM server key and 'device_fcm_token'
with the FCM token of the device you want to send the notification to. You can customize the title, body, and extra parameters as needed. This script sends an FCM notification with the specified parameters.
What's Your Reaction?
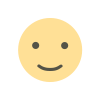
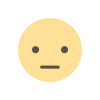
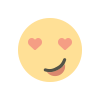
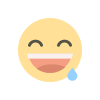
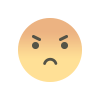
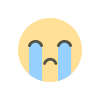
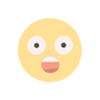